To retrieve a list of hosts using the Cisco DNA Center API, the provided Python script needs to be completed with the appropriate code snippets. Here's how the final script looks:
import json, requests, urllib3
from requests.auth import HTTPBasicAuth
from config import host, username, password
headers = { 'Content-Type': "application/json", 'x-auth-token': "csd0934rjxx" }
def dna_api_auth(host, username, password):
url = "https://{}/dna/system/api/v1/auth/token ".format(host)
response = requests.post(url, auth=HTTPBasicAuth(username, password), headers=headers, verify=False)
token = response.json()["Token"]
return token
def list_dna_devices(token):
url = "https://{}/dna/intent/api/v1/network-device ".format(host)
headers["x-auth-token"] = token
response = requests.get(url, headers=headers, verify=False)
data = response.json()
for item in data['response']:
print(item["hostname"])
token = dna_api_auth(host, username, password)
list_dna_devices(token)
application/json: This is used to set the content type for the headers.
token = response.json()["Token"]: This extracts the authentication token from the response.
data['response']: This accesses the 'response' part of the JSON data returned by the API.
token: This passes the token to the headers for authorization.
json(): This parses the response to JSON format.
for item in data['response']: This loops through each item in the response data.
hostname: This prints the hostname of each device.
References:
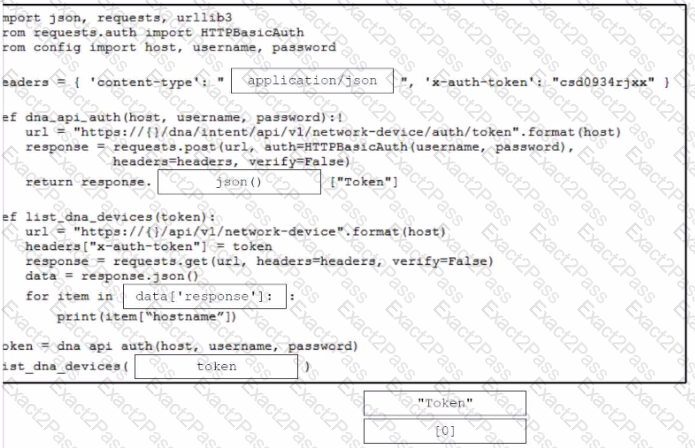